What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. It is often used to break complex problems into smaller, more manageable subproblems.
How Recursion Works in JavaScript
A recursive function in JavaScript typically has two main components:
Base Case: This is the condition that stops the recursion to prevent an infinite loop.
Recursive Case: This is where the function calls itself with a modified argument, gradually moving toward the base case recursion javascript.
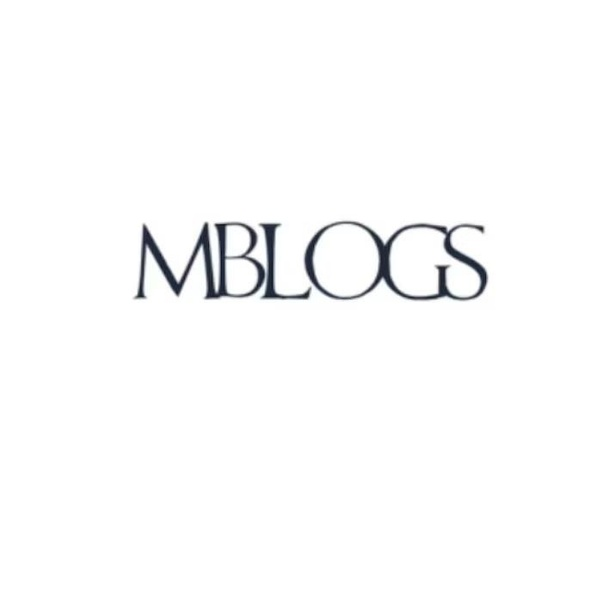
Benefits of Using Recursion
Simplifies complex problems like tree traversal, backtracking, and divide-and-conquer algorithms.
Reduces the need for loops, making code more readable in certain scenarios.
Useful for mathematical problems like calculating factorial, Fibonacci sequences, and permutations.
Challenges of Recursion
Risk of infinite recursion if the base case is not properly defined.
Consumes more memory due to the function call stack, leading to potential stack overflow errors.
Can be less efficient than iterative approaches in some cases.
Common Use Cases of Recursion
Tree and Graph Traversal: Searching and modifying tree-like structures efficiently.
Sorting Algorithms: QuickSort and MergeSort use recursion for efficient sorting.
Mathematical Computations: Factorials, Fibonacci sequences, and exponentiation.
Conclusion
Recursion is a powerful tool in JavaScript that helps in solving problems that require breaking down tasks into smaller instances. However, it should be used carefully to avoid performance issues and stack overflows. Understanding when to use recursion can make your JavaScript programming more efficient and elegant.